Form
This module adds the ability for users to create and share forms.
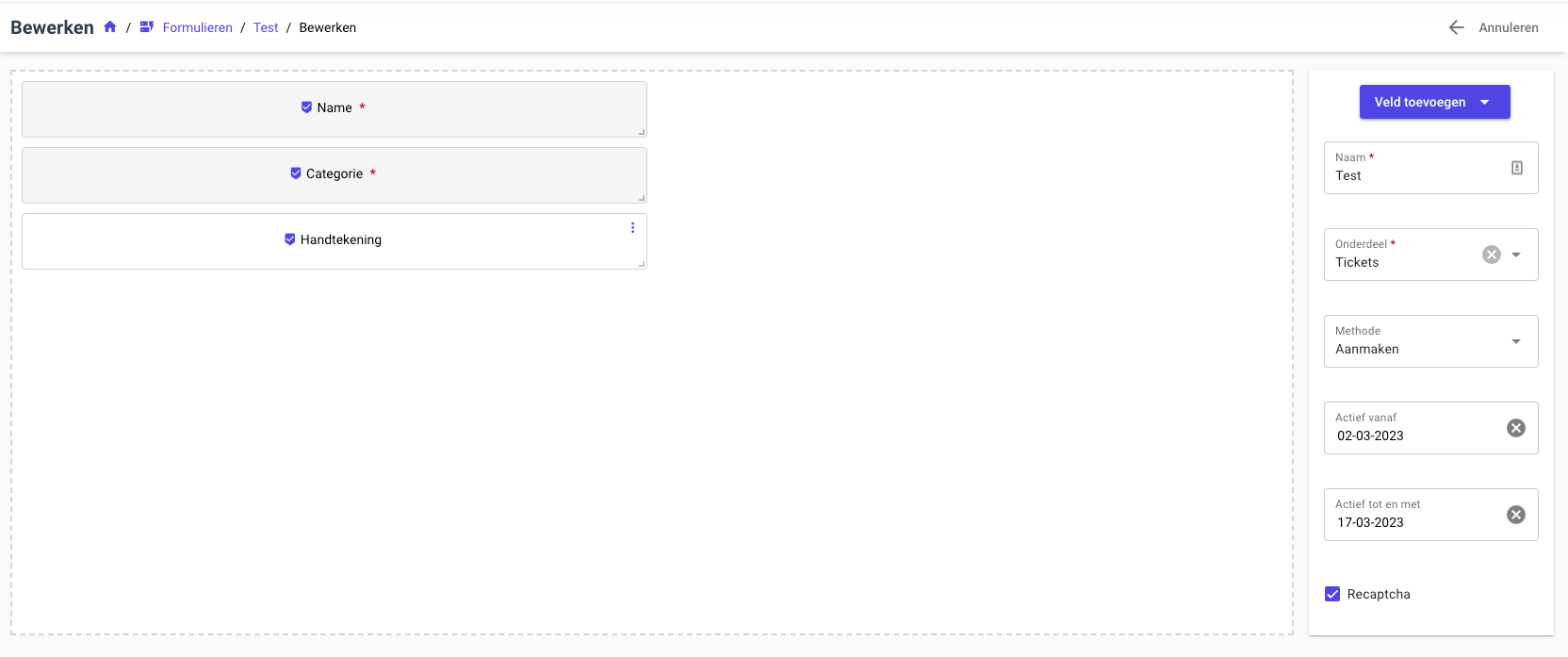
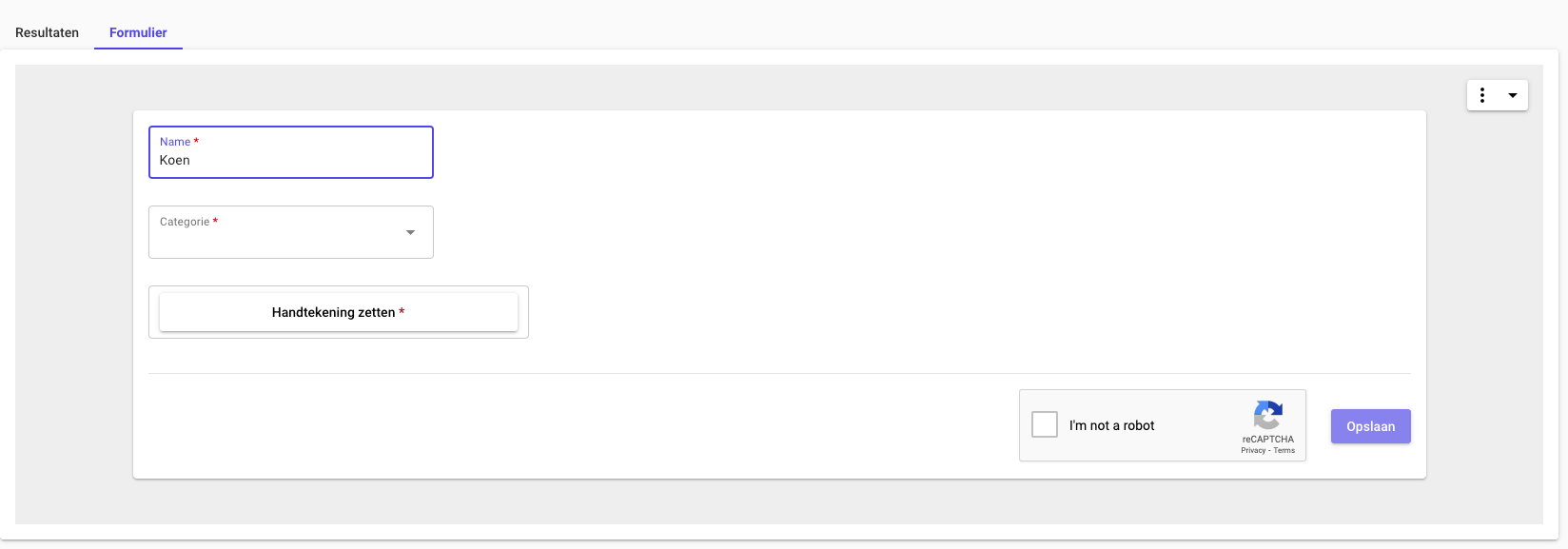
Installation
composer require qore/forms
php artisan vendor:publish --tag=qore.forms.db
php artisan vendor:publish --tag=qore.forms.frontend
Route
Created forms can be shared publicly. You will have to expose your own route for this.
Add the following route to frontend/src/router/routes.js
(at the end, outside of middleware, but just before the *
route):
// HERE:
{ path: '/public-form/:uuid', name: 'publicForm', component: () => import('../vendor/forms/Pages/PublicForm.vue') }
// Always leave this as last one,
// but you can also remove it
{
path: '*',
component: () => import('pages/error/Error404.vue')
}
Recaptcha
Recaptcha is used to prevent bots from submitting forms. By default, Recaptcha v2 is used, and an API key by Koen is configured. You can override this by adding the following to your back-end .env:
RECAPTCHA_SITE_KEY=
RECAPTCHA_SECRET=
Usage
Forms will create links that exists in the central database. Make sure to run all migrations:
php artisan migrate
php artisan tenants:migrate
- Forms exists per tenant in the
forms
table - Form results (submitted forms) exists per tenant in the
form_results
andform_result_values
tables - Form link exists centrally in the
form_links
table
Add to menu
After enabling the module, you can add the following to GlobalsController
:
if (module_is_active('qore/forms')) {
$tab->addResourceMenuItem(resource(FormResource::class));
}
Event handling
There are 2 events that will be emitted. The first one will be emitted when a user has submitted a form, and the second one when the FormResult has been created. You can add your own listeners for these if needed:
CustomFormSubmitted::class
FormResultCreated::class
Configuration overrides
You can override the forms.php
configuration file:
php artisan vendor:publish --tag=qore.forms.config
Mailing
In some cases you might want to send form links in bulk to clients / users. In order to do this, the follow module needs to be installed: Mailing extended.
After this is installed and enabled, a mail list needs to be created.
Finally, a mail template & variable list should be created (See documentation).
The following tag should be included in your VariableList
and mail template content:
{{ form_url }}
Your variable list should look something like this:
class TicketVariableList extends VariableList
{
public function variables(): array
{
return [
__('Variables') => [
'form_url'
]
];
}
public function name(): string
{
return 'Client variable list';
}
public function resource(): QoreResource
{
return resource('clients');
}
public function map(?int $modelId, array $args = []): array
{
$model = Ticket::find($modelId);
$form = Form::find($args['form_id']);
$formUrl = null;
if ($form) {
$link = FormUtility::makeLink($form, $model->id);
$formUrl = '<a href="' . $link->getUrl() . '">Open the form!</a>';
}
return [
'form_url' => $formUrl
];
}
}
When sending the mail manually, make sure to pass along the form_id
:
$mailList = resource('mail_lists')->retrieve($arguments['state']['mail_list']);
$to = $mailList->getFilteredModels()->pluck('id')->toArray();
$mailTemplate = MailTemplate::find($arguments['state']['mail_template']);
(new BulkMessageMailer(
$mailTemplate,
$to,
['form_id' => $this->model->id],
null,
[],
$this->model
))->send();
Upgrade Guide
composer update qore/forms
If you need to upgrade migrations or Vue components:
php artisan vendor:publish --tag=qore.forms.db --force
php artisan vendor:publish --tag=qore.forms.frontend --force